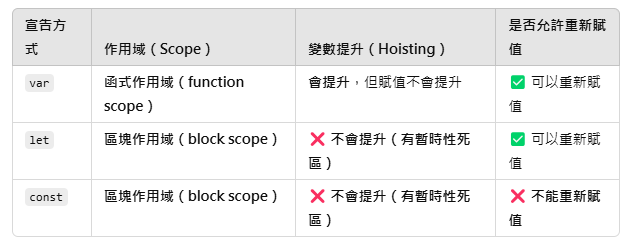
function scope() {
foo = 1; // ❗ 未宣告的變數,會變成全域變數(非嚴格模式)
}
scope();
console.log(foo); // ✅ 1 (`foo` 變成全域變數)
"use strict";
function scope() {
foo = 1; // ❌ ReferenceError: foo is not defined
}
scope();
var
function scope() {
foo = 1; // ❓ 這裡的 `foo` 的 scope 是什麼?
var foo;
}
scope();
function scope() {
var foo; // 變數提升 (Hoisting)
foo = 1; // 在函式作用域內賦值
}
function scope
function testFunctionScope() {
if (true) {
var foo = "hello";
}
console.log(foo); // ✅ "hello"(`var` 被提升到整個函式範圍)
}
testFunctionScope();
console.log(foo); // ❌ ReferenceError: foo is not defined(函式外無法存取)
const
function scope() {
foo = 1; // ❌ ReferenceError: Cannot access 'foo' before initialization
const foo;
}
scope();
let
function scope() {
foo = 1; // ❓ ReferenceError: Cannot access 'foo' before initialization
let foo;
}
scope();
block scope
function testBlockScope() {
if (true) {
let foo = "hello";
const bar = "world";
console.log(foo); // ✅ "hello"
console.log(bar); // ✅ "world"
}
console.log(foo); // ❌ ReferenceError: foo is not defined
console.log(bar); // ❌ ReferenceError: bar is not defined
}
testBlockScope();