I have a Next.js website application that uses a MongoDB database, and I am developing it using Docker containers. The original system was intended to be deployed on a NAS after development, and the NAS system is an ARM32 platform, not an Intel platform. As a result, the original program needs to be modified to support ARM32. The application’s image was changed from node:lts-alpine
to arm32v7/node:lts-alpine
, and the database image was changed from mongo:3.6.23
to apcheamitru/arm32v7-mongo:latest
.
Local Docker Development
If you are not familiar Docker, please refer to
If you are not familiar with Next.js, please refer to these articles.
Here is the Docker-intel, use the command to generate a Docker image.
docker build -f Dockerfile-intel -t fluber/wood:latest . Reference: Docker-Hub.
FROM node:lts-alpine
# 設置工作目錄
WORKDIR /home/node/code/my-wood-co2-app
# 複製 package.json 和 package-lock.json
COPY ./code/test ./
# 安裝依賴(可選:加快安裝過程)
RUN npm ci || npm install
# 設置環境變數
ENV HOST=0.0.0.0
ENV CHOKIDAR_USEPOLLING=true
ENV CHOKIDAR_INTERVAL=100
# 開放應用使用的端口
EXPOSE 3001
# 啟動應用
CMD ["npm", "run", "start"]
Here is the Docker-arm32v7, use the command to generate a Docker image:.
docker build -f Dockerfile-arm32v7 -t fluber/wood:wood:armv7 .
# 使用 arm32v7 版本的 Node.js LTS Alpine 映像
FROM arm32v7/node:lts-alpine
# 設置工作目錄
WORKDIR /home/node/code/my-wood-co3-app
# 複製 package.json 和 package-lock.json
COPY ./code/arm32_test ./
# 安裝依賴(可選:加快安裝過程)
RUN npm ci || npm install
# 確保在正確的目錄中運行 build 步驟
WORKDIR /home/node/code/my-wood-co3-app
# 設置環境變數
ENV HOST=0.0.0.0
ENV CHOKIDAR_USEPOLLING=true
ENV CHOKIDAR_INTERVAL=100
# 開放應用使用的端口
EXPOSE 80
# 啟動應用
CMD ["npm", "run", "start"]
Here is the docker-compose-intel-production.yml
services:
application:
container_name: my-wood-co2-application
image: fluber/wood:latest
ports:
- "3001:3001"
working_dir: /home/node/code/my-wood-co2-app
environment:
- HOST=0.0.0.0
- CHOKIDAR_USEPOLLING=true
- CHOKIDAR_INTERVAL=100
- MONGO_URI=mongodb://admin:secretpassword@mongodb:27017/wood_co2_db?authSource=admin
tty: true
depends_on:
- mongodb
mongodb:
container_name: my-mongodb
image: mongo:3.6.23
restart: always
ports:
- "27017:27017"
volumes:
- mongodb_data:/data/db
environment:
- MONGO_INITDB_ROOT_USERNAME=admin
- MONGO_INITDB_ROOT_PASSWORD=secretpassword
- MONGO_INITDB_DATABASE=wood_co2_db
healthcheck:
test: ["CMD", "mongo", "--username", "admin", "--password", "secretpassword", "--eval", "db.adminCommand('ping')"]
interval: 30s
retries: 3
start_period: 10s
timeout: 5s
mongo-express:
container_name: my-mongo-express
image: mongo-express
restart: always
ports:
- "8081:8081"
environment:
- ME_CONFIG_MONGODB_ADMINUSERNAME=admin
- ME_CONFIG_MONGODB_ADMINPASSWORD=secretpassword
- ME_CONFIG_MONGODB_SERVER=my-mongodb
- ME_CONFIG_BASICAUTH=false # Disable login authentication
depends_on:
- mongodb
volumes:
mongodb_data:
Here is the docker-compose-arm32-production.yml
services:
application:
container_name: my-wood-co2-application
image: fluber/wood:armv7
ports:
- "80:80"
working_dir: /home/node/code/my-wood-co3-app
environment:
- HOST=0.0.0.0
- CHOKIDAR_USEPOLLING=true
- CHOKIDAR_INTERVAL=100
- MONGO_URI=mongodb://admin:secretpassword@mongodb:27017/wood_co2_db?authSource=admin
tty: true
depends_on:
- mongodb
mongodb:
container_name: my-mongodb
#image: mongo:latest
image: apcheamitru/arm32v7-mongo:latest
restart: always
ports:
- "27017:27017"
volumes:
- mongodb_data:/data/db
environment:
- MONGO_INITDB_ROOT_USERNAME=admin
- MONGO_INITDB_ROOT_PASSWORD=secretpassword
- MONGO_INITDB_DATABASE=wood_co2_db
healthcheck:
test: ["CMD", "mongo", "--username", "admin", "--password", "secretpassword", "--eval", "db.adminCommand('ping')"]
interval: 30s
retries: 3
start_period: 10s
timeout: 5s
volumes:
mongodb_data:
λ docker compose -f docker-compose-intel-production.yml up -d
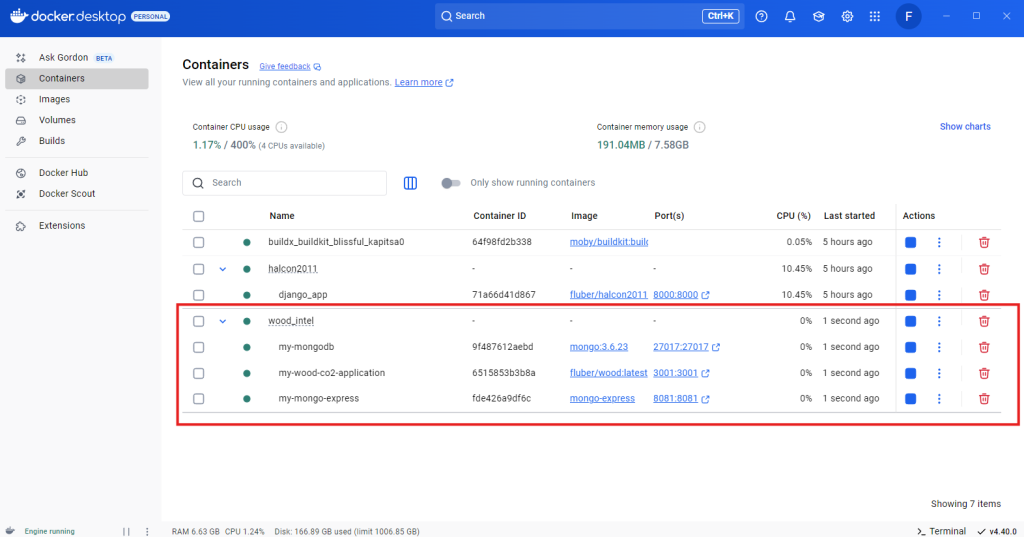
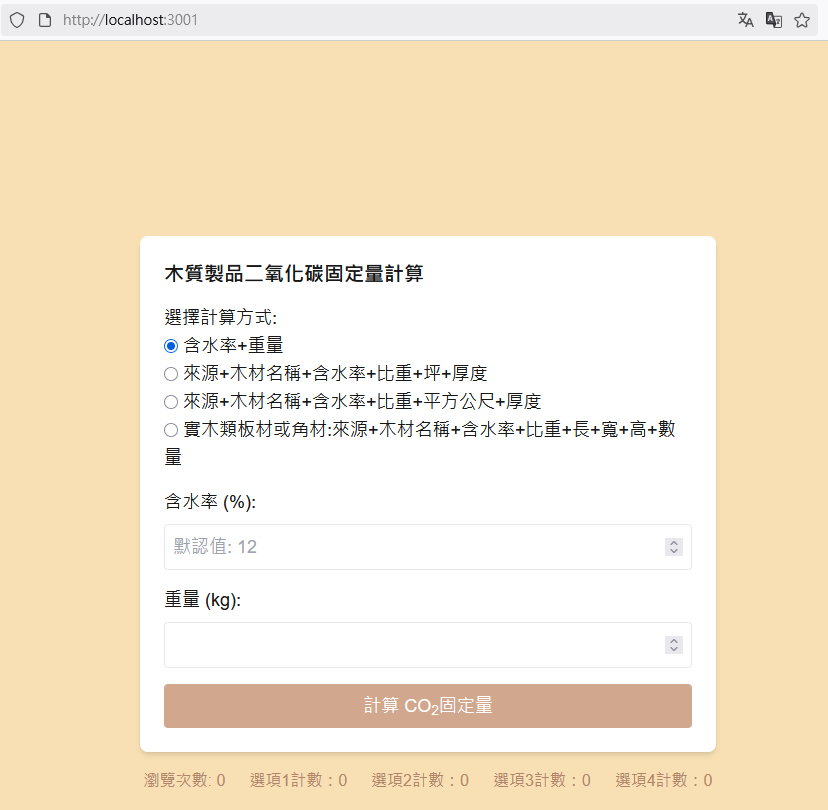
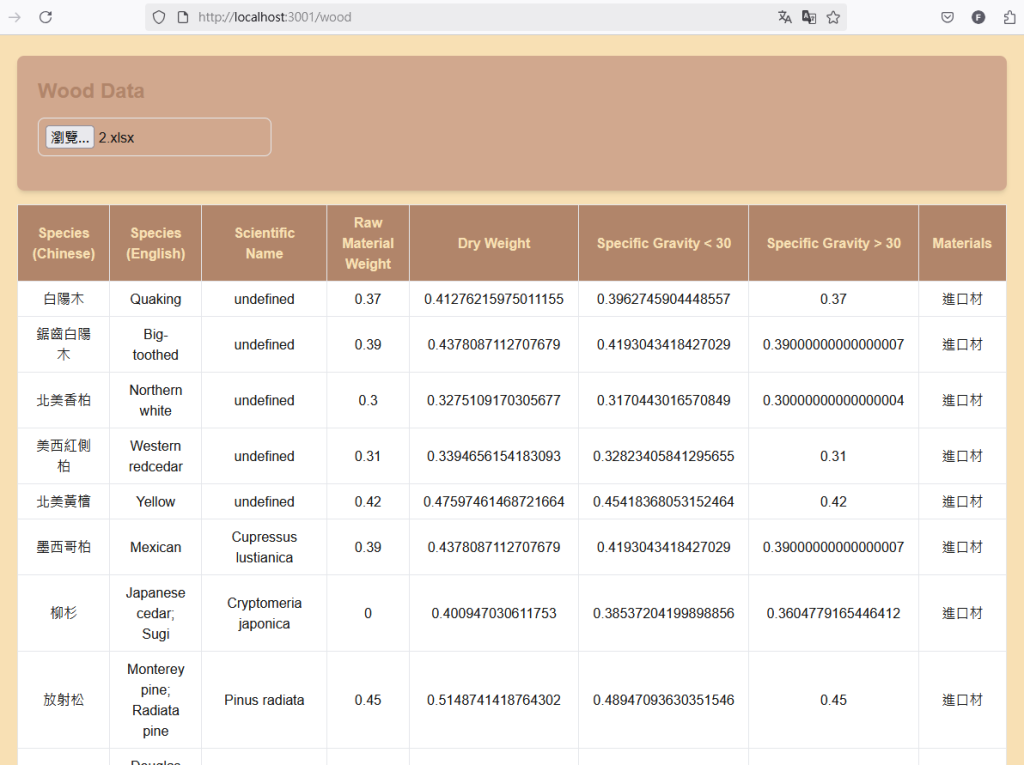
Prepare to deploy to Google Cloud Run
- Use an external MongoDB service to replace the mongodb block in docker-compose.yml
- You can use a Google account to activate Google Cloud services, which requires your credit card information.
- You need to remove the MongoDB and mongo-express sections from the
docker-compose.yml
file and add theMONGO_URI
environment variable to the application section to connect to an external MongoDB database. - Create a Google Cloud Project, and the system will provide a project ID, which will be used later.
- Install the gcloud CLI if you haven’t: https://cloud.google.com/sdk/docs/install
- Tag & Push Docker Image
- Deploy to Cloud Run
Apply for MongoDB Atlas
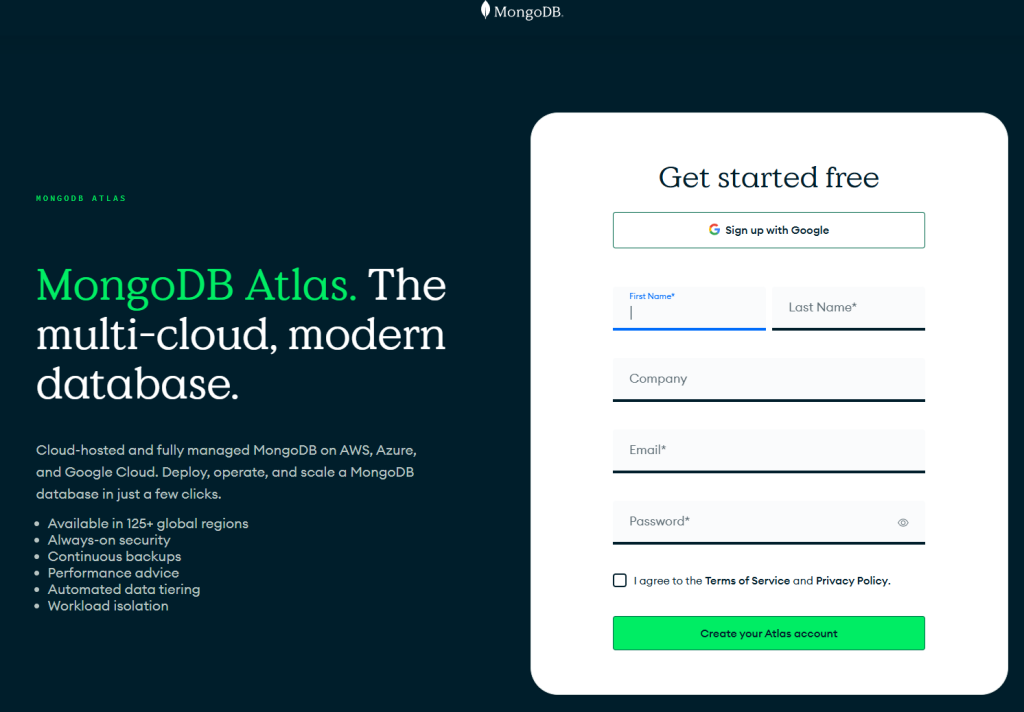
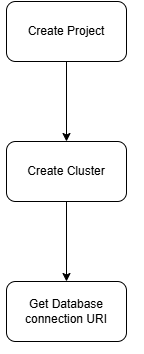
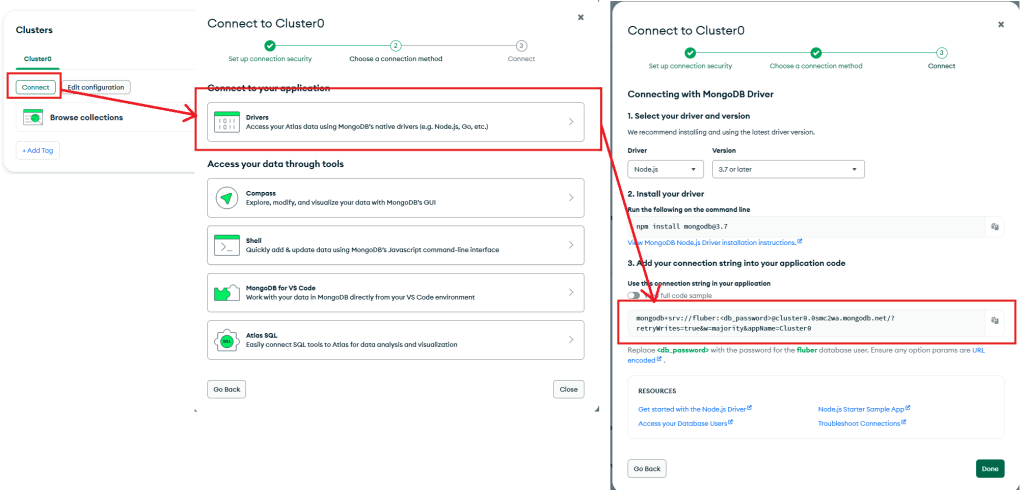
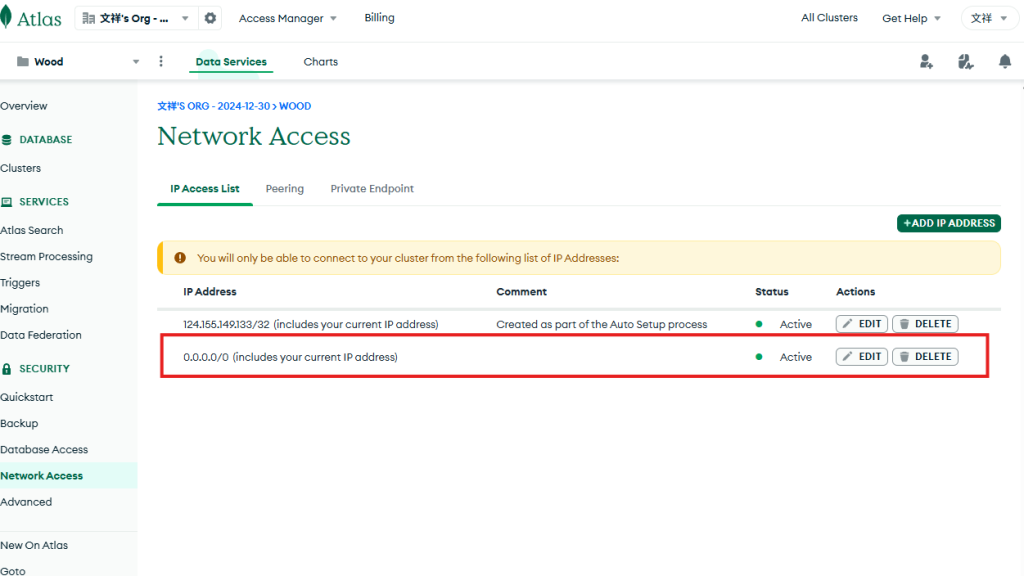
For temporary testing, you can do it this way.
Create a Cloud Project
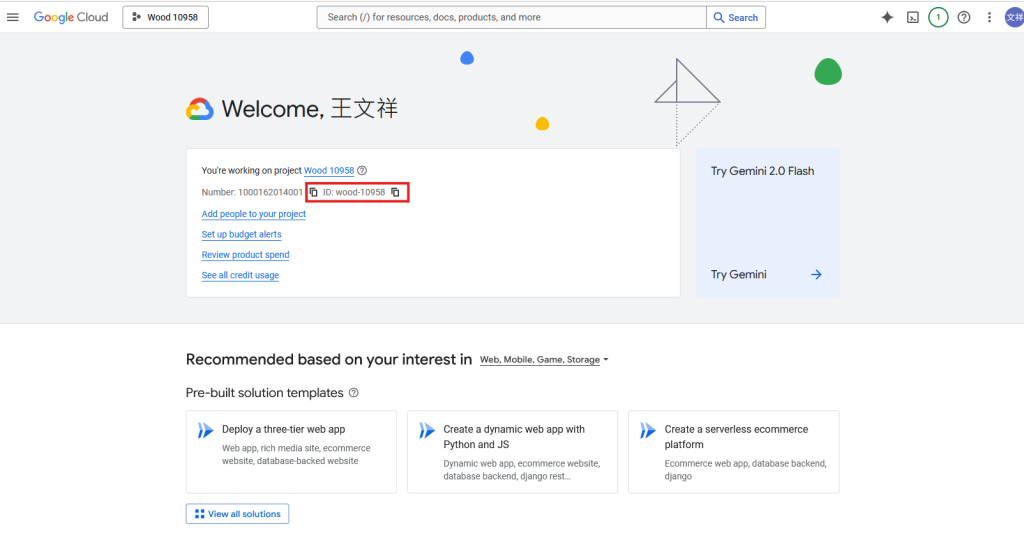
gcloud auth login
gcloud config set project wood-10958
gcloud auth configure-docker
# Tag the image for GCR
docker tag fluber/wood:latest gcr.io/wood-10958/wood-app
# Push it
docker push gcr.io/wood-10958/wood-app
#Try Using Artifact Registry Instead (Recommended)
gcloud services enable artifactregistry.googleapis.com
#Create a Docker Repository
gcloud artifacts repositories create wood-repo \
--repository-format=docker \
--location=us-central1 \
--description="Docker repo for Wood app"
#Tag & Push to Artifact Registry
docker tag fluber/wood:latest us-central1-docker.pkg.dev/wood-10958/wood-repo/wood-app:latest
docker push us-central1-docker.pkg.dev/wood-10958/wood-repo/wood-app:latest
#Re-authenticate and Configure Docker
gcloud auth configure-docker us-central1-docker.pkg.dev
#Try Pushing Again
docker push us-central1-docker.pkg.dev/wood-10958/wood-repo/wood-app:latest
gcloud run deploy wood-app \
--image=us-central1-docker.pkg.dev/wood-10958/wood-repo/wood-app:latest \
--platform=managed \
--region=us-central1 \
--allow-unauthenticated \
--port=3001 \
--set-env-vars=HOST=0.0.0.0,CHOKIDAR_USEPOLLING=true,CHOKIDAR_INTERVAL=100,MONGODB_URI=mongodb+srv://fluber:<db_password>@cluster0.0smc2wa.mongodb.net/?retryWrites=true&w=majority&appName=Cluster0
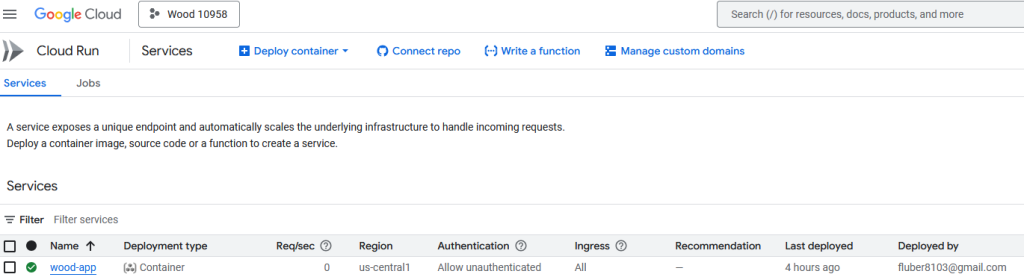
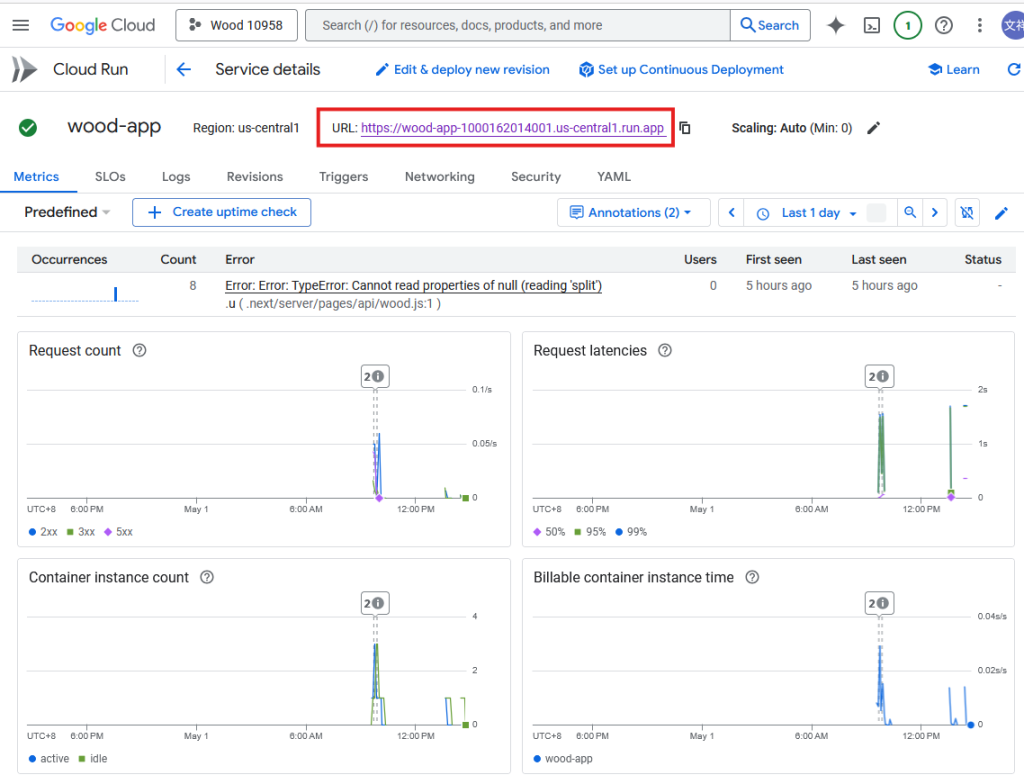
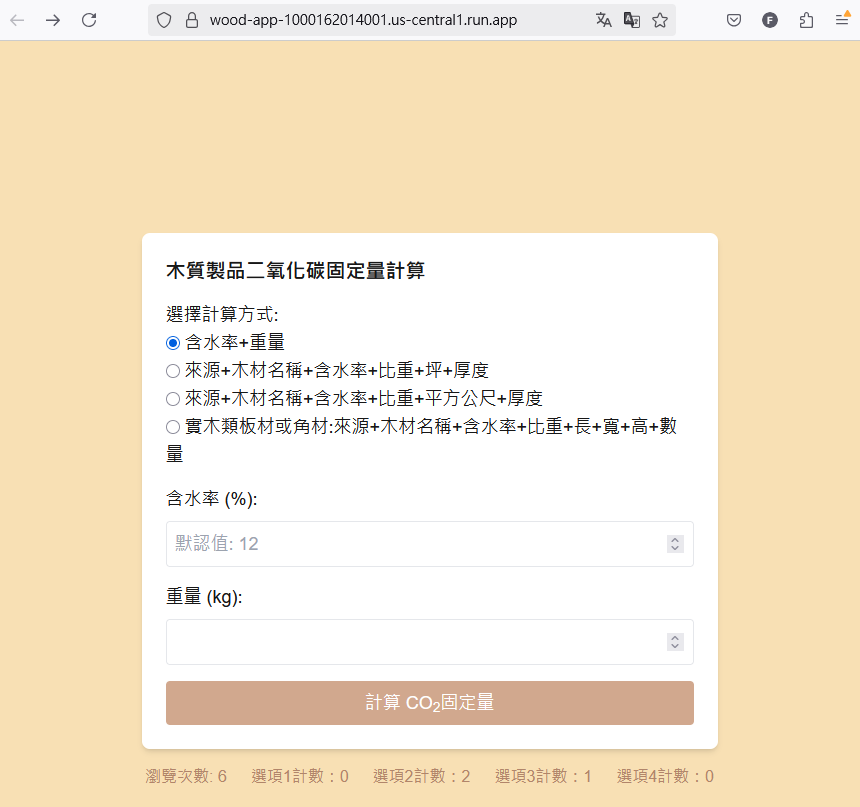
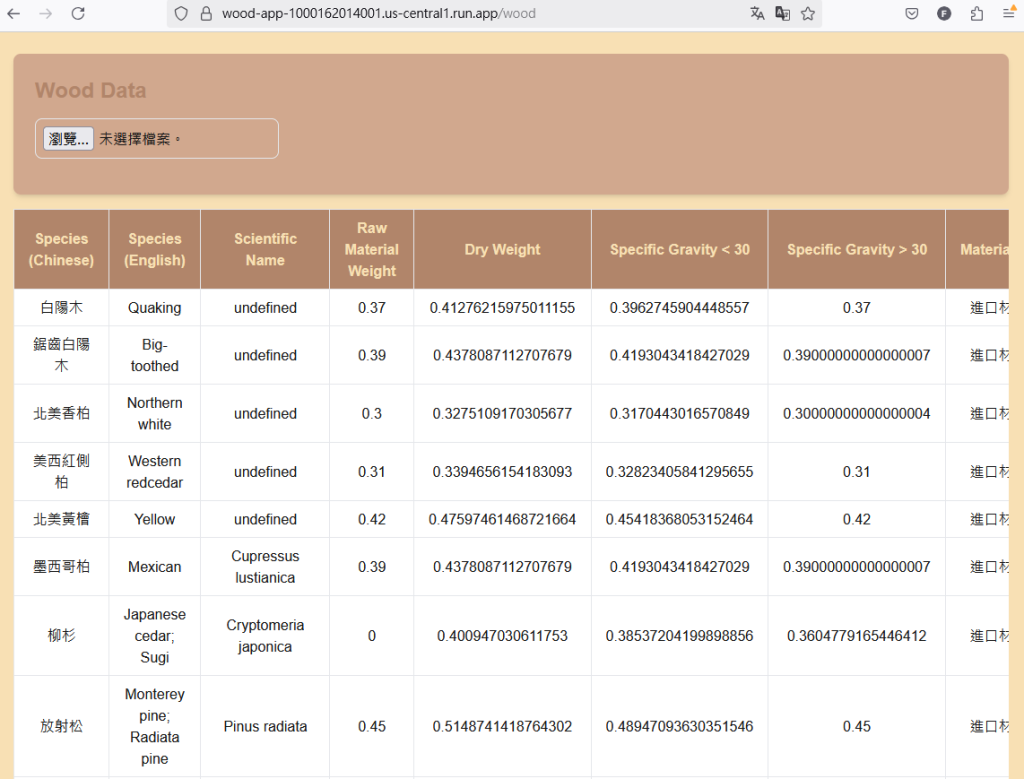
Google Cloud Run vs. AWS App Runner
In the chart, each column (IaaS, STaaS, PaaS, DaaS, FaaS, SaaS) represents a cloud service model, and each row (e.g., Datacenters, Networking, Storage, etc.) represents a layer of the technology stack needed to deliver an application.
- Gray-colored boxes indicate:
🔧 Managed by the Cloud Provider (e.g., AWS)
→ You do not have to manage these components yourself. - White-colored boxes indicate:
👤 Managed by You (the customer/developer)
→ You are responsible for configuring, maintaining, or developing on that layer.
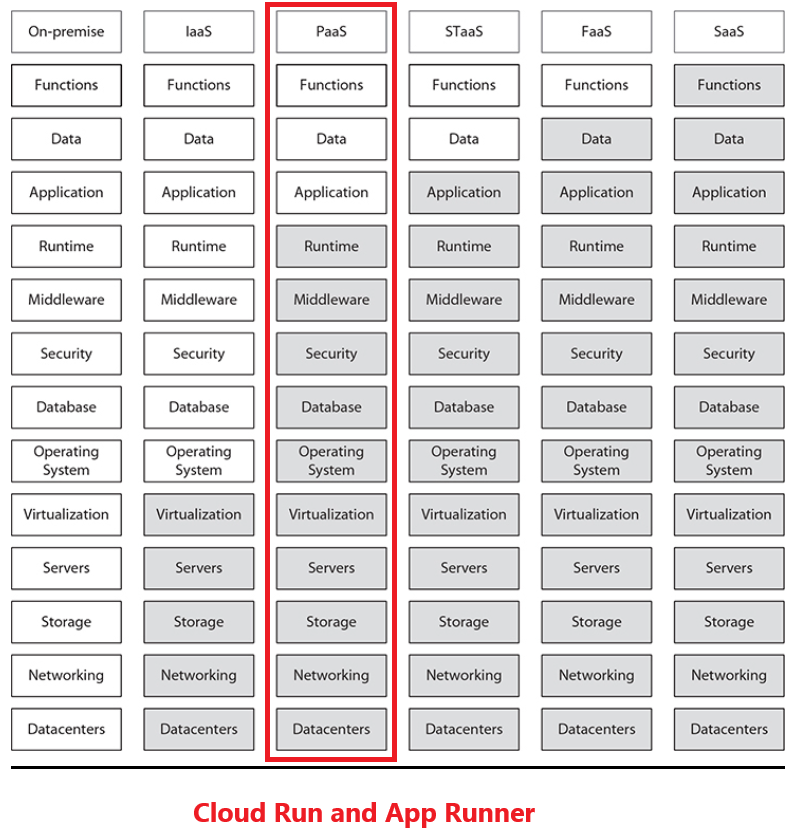
Why Google Cloud Run and AWS App Runner are PaaS (Platform as a Service):
- No Infrastructure Management:
You don’t need to manage servers or hardware. You just upload your application (usually in a container), and the platform takes care of running it for you. - Focus on Code:
You focus on writing your application code. The platform handles everything else like scaling, networking, and managing servers. - Automatic Scaling:
Both platforms automatically adjust the number of resources (like containers) based on traffic. You don’t need to worry about how many servers you need or when to add more. - Managed Services:
They handle all the technical details for you, like monitoring, logging, and updating the system. You don’t have to deal with any of that. - Simple Deployment:
You can easily deploy your code, often with tools that automate the process. You don’t need to worry about setting up servers or handling complex configurations. - No Server Worries:
These platforms take care of things like server updates and maintenance. You don’t need to worry about keeping servers running or making sure they are up to date.
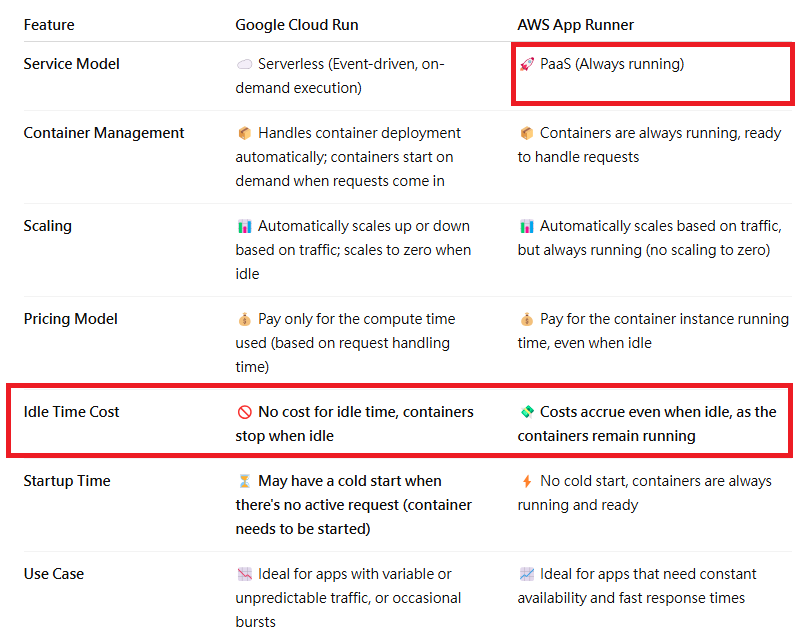
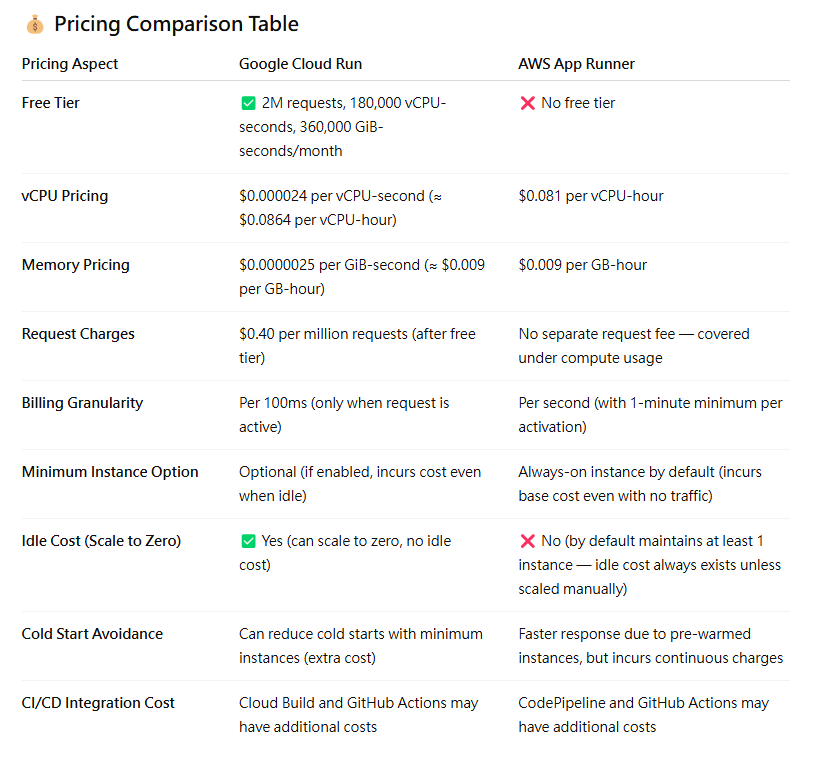