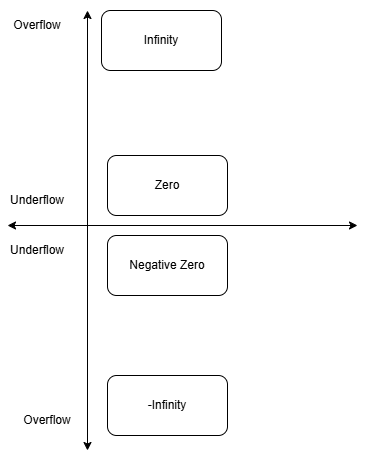
- Infinity: A number operation is larger than the largest representable number. (Overflow)
- -Infinity: Similarly, when the absolute value of a negative value becomes larger than the absolute value of the largest representable negative number. (Overflow)
- Zero: when the result of a numeric operation is closer to zero than the smallest representable number. (Underflow)
- negative zero: if the underflow occurs from a negative number
1 / 0 // => Infinity
Number.MAX_VALUE * 2 // => Infinity
-1 / 0 // => -Infinity
-Number.MAX_VALUE * 2 // => -Infinity
Not-a-number, NaN
- zero divided by zero
- infinity divided by infinity
- take the square root of a negative number
- use arithmetic operators with no-numeric operands thant cannot be converted to numbers
0 / 0 // => NaN
Infinity / Infinity // => NaN
isNaN(x) // to determine whether the value of a variable x is NaN
Number.isNaN(x) // to determine whether the value of a variable x is NaN
Binary floating-point numbers (IEEE-754)
let x = .3 - .2; //console.log(0.3 - 0.2); // 0.09999999999999998
let y = .2 - .1; //console.log(0.2 - 0.1); // 0.1
x === y // => false: because 0.09999999999999998 !== 0.1
x === .1 // => false: .3 - .2 is not equal to .1
y === .1 // => true: .2 - .1 is equal to .-1
✅ Solution: Use Number.EPSILON
for Comparison
console.log(Math.abs(x - y) < Number.EPSILON); // true ✅
function areAlmostEqual(a, b) {
return Math.abs(a - b) < 1e-10; // Choose a small tolerance
}
console.log(areAlmostEqual(x, y)); // true ✅
BigInt
BigInt
is a special data type in JavaScript used to store and manipulate large integers beyond the Number
type’s limit (2^53 - 1
).
JavaScript’s Number
type uses 64-bit floating-point representation, meaning:
- The largest safe integer is
2^53 - 1
(9007199254740991
). - Going beyond this limit can cause precision errors.
console.log(9007199254740991 + 1); // 9007199254740992 ✅
console.log(9007199254740991 + 2); // 9007199254740992 ❌ (Wrong!)
let bigNumber = 9007199254740991n; // Add "n" at the end
console.log(bigNumber + 2n); // ✅ Works correctly!
1 < 2n // => true
2 > 1n // => true
0 == 0n // => true
0 === 0n // => false, the === checks for type equality as well
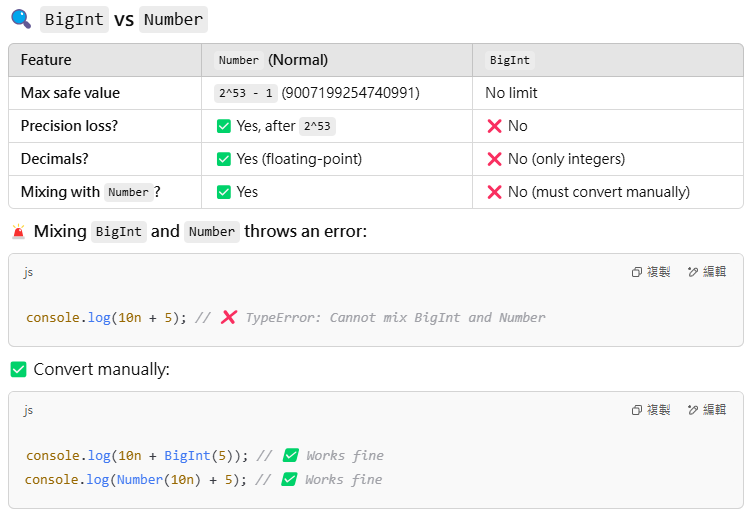
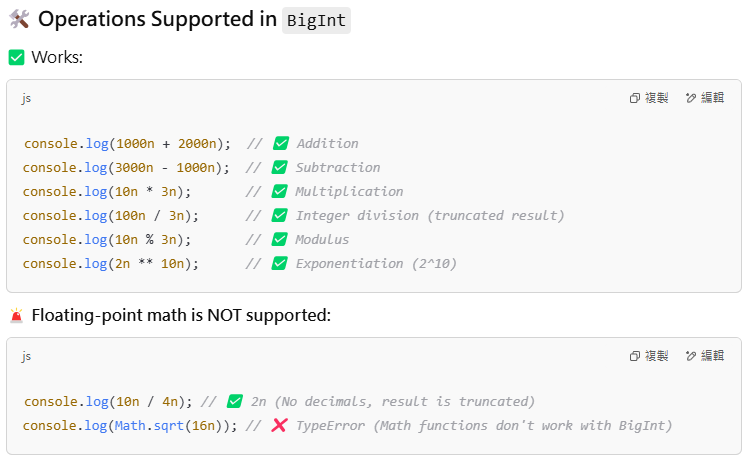