Javascript not only a script language, it is a efficient general-purpose language suitable for software engineering and projects with huge codebases.
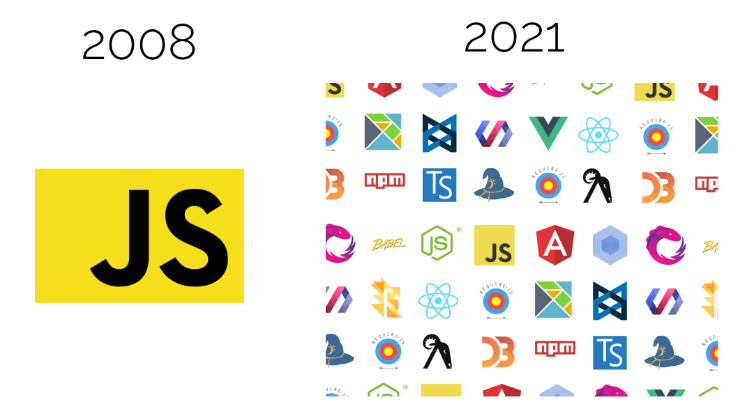
JavaScript was invented by Brendan Eich in 1995.
It was developed for Netscape 2, and became the ECMA-262 standard in 1997.
After Netscape handed JavaScript over to ECMA, the Mozilla foundation continued to develop JavaScript for the Firefox browser. Mozilla’s latest version was 1.8.5. (Identical to ES5).
ECMA-the European Computer Manufacture Association, JavaScript is also known as EMCAScript. For most of the 2010s, version 5 of the ECMAScript standard has been supported by all web browsers. This book treats ES5 as the compatibility baseline and no longer discusses earlier versions of the language. ES6 was released in 2015 and added major new features—including class and module syntax—that changed JavaScript from a scripting language into a serious, general-purpose language suitable for large-scale software engineering.
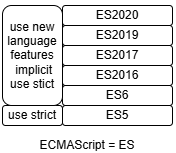
As JavaScript evolved, the language designers attempted to correct flaws in the early (pre-ES5) versions. In order to maintain backward compatibility, it is not possible to remove legacy features, no matter how flawed. But in ES5 and later, programs can opt in to JavaScript’s strict mode in which a number of early language mistakes have been corrected. The mechanism for opting in is the “use strict” directive
In ES6 and later, the use of new language features often implicitly invokes strict mode. For example, if you use the ES6 class keyword or create an ES6 module, then all the code within the class or module is automatically strict.
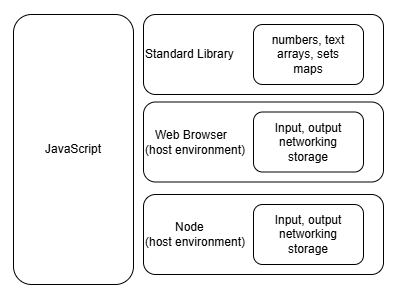
The JavaScript Standard Library just include apis for numbers, text, arrays, sets, and maps, It doesn’t include the Input/Ouput, networking, storage, graphics… apis, these apis are provided by host environments, web browers and Node.
A Tour of JavaScript
- // Anything folling double slashes is comment
- let x; // Variable is declared with let keyword, declare a variable named x
- x = 100; // Value can be assigned to a variable with = sign
- x // A variable can be evaluates to its value
- supoort several types of values, number, string, boolean, null, undefined
- null is a special value that means “no value”
- undefined is another special value like null.
- declare a object, objects are enclosed in curly braces.
let car = {
brand: "Toyota",
year_of_manufacture: 2025
};
- car.brand // Access the property of an object with .
- car[“brand”] // Access the property of an object with [ ]
- car.price = 100000; // Create a new property by assignment
- car.engine = {}; // assign an empty object with no properties using {}
- car.engine?.horsepower // Conditionally access properties with ?.
- JavaScript also supports arrays (numerically indexed lists) of values
- Arrays and objects can hold other arrays and objects
let apples = [20, 30, 50, 70];
let points = [
{x: 100, y: 100},
{x: 200, y: 100}
];
let rectangle = {
line1: [[100, 100], [200, 100]],
line2: [[100, 200], [200, 200]],
line3: [[100, 100], [100, 200]],
line4: [[200, 100], [200, 200]]
}
- Operators act on values (the operands) to produce a new value.
30 + 20 // => 50: addition
30 - 20 // => 10: subtraction
30 * 20 // => 600: multiplication
30 / 20 // => 1.5: division
rectangle.line4[0][0] - rectangle.line1[0][0] // => 100: more complicated operands also work
"30" + "20" // => "3020": concatenates strings
- JavaScript defines some shorthand arithmetic operators
let money = 100; // Define a variable, the initial value is 100
money++; // Increment the variable
money--; // Decrement the variable
money += 20; // Add 20: same as money = money + 20;
money *= 30; // Multiply by 30; same as money = money * 30;
money; // => 3600: variable names are expression, too.
- Equality and relational operators test whether two values are equal. unequal, less than, greater than…
let x = 20, y = 30; // These = sign are assigment
x === y // => false: equality
x !== y // => true: inequality
x < y // => true: less-than
x <= y // => true: less-than or equal
x > y // => false: greater-than
x >= y // => false: greater-than or equal
"two" === "three" // => false: two strings are different
"two" > "three" // => true: "tw" is alphabetically greater than "th"
false === (x > y) // => true: false is equal to false
(x === 20) && (y === 30) // => true: both comparisons are true
(x > 3) || (y < 3) // => false: neither comparison is true
!(x === y) // => true: inverts a boolean value
- functions are parameterized blocks of JavaScript code that we can invoke
function plus100(x) {
return x + 100;
}
let triple = function(x) {
return x * x * x;
}
triple(plus100(100))
- This concise syntax use => to separate the argument list from the function body we call the Arrow function
const plus100 = x => x + 100;
const triple = x => x * x * x;
triple(plus100(100));
- control structures, if, for, and while
if (x > 0) {
......
} else {
......
}
for (let x of array) {
.....
}
while (n > 1) {
.....
}
- declare a class
class Rectangle {
constructor(x, y, width, height) {
this.x = y;
this.y = y;
this.width = width;
this.height = height;
}
area() {
return this.width * this.height;
}
}
let roi = new Recangle(100, 100, 200, 300);
roi.area() // => 60000