Make sure you next.config.js content
//next/config.js
const createNextIntlPlugin = require('next-intl/plugin');
const withNextIntl = createNextIntlPlugin();
/** @type {import('next').NextConfig} */
const nextConfig = {
webpack: (config, { buildId, dev, isServer, defaultLoaders, webpack }) => {
// 添加 PDF 加載規則
config.module.rules.push({
test: /\.pdf$/,
use: [
{
loader: 'file-loader',
options: {
name: '[path][name].[ext]',
},
},
{
loader: 'pdf-loader',
},
],
});
return config;
},
};
// 使用 `withNextIntl` 包裹 nextConfig
module.exports = withNextIntl(nextConfig);
To Build Next.js Source Code and Start for check
/home/node/code/nextjs-template # ls
LICENSE messages next.config.js.old.1 postcss.config.js tailwind.config.ts
README.md next-env.d.ts node_modules public tsconfig.json
components.json next.config.js package-lock.json public.zip yarn.lock
lib next.config.js.old package.json src
/home/node/code/nextjs-template # rm -rf .next
/home/node/code/nextjs-template # npm run build
> next-template@0.1.0 build
> next build
Attention: Next.js now collects completely anonymous telemetry regarding usage.
This information is used to shape Next.js' roadmap and prioritize features.
You can learn more, including how to opt-out if you'd not like to participate in this anonymous program, by visiting the following URL:
https://nextjs.org/telemetry
▲ Next.js 14.2.24
- Environments: .env.local
Creating an optimized production build ...
(node:8225) [DEP0040] DeprecationWarning: The `punycode` module is deprecated. Please use a userland alternative instead.
(Use `node --trace-deprecation ...` to show where the warning was created)
✓ Compiled successfully
✓ Linting and checking validity of types
✓ Collecting page data
✓ Generating static pages (5/5)
✓ Collecting build traces
✓ Finalizing page optimization
Route (app) Size First Load JS
┌ ○ /_not-found 873 B 88.5 kB
├ ƒ /[locale] 1.43 kB 109 kB
├ ƒ /[locale]/about 1.29 kB 104 kB
├ ƒ /[locale]/contact 1.83 kB 105 kB
├ ƒ /[locale]/portfolio 2.12 kB 117 kB
├ ƒ /[locale]/support 377 B 103 kB
└ ƒ /api/contact 0 B 0 B
+ First Load JS shared by all 87.6 kB
├ chunks/117-82d3a646c049e5a6.js 31.6 kB
├ chunks/fd9d1056-48f7f5602f8e2d67.js 53.6 kB
└ other shared chunks (total) 2.42 kB
ƒ Middleware 51.1 kB
○ (Static) prerendered as static content
ƒ (Dynamic) server-rendered on demand
/home/node/code/nextjs-template # npm run start
> next-template@0.1.0 start
> next start
▲ Next.js 14.2.24
- Local: http://localhost:3000
✓ Starting...
✓ Ready in 4.8s
Copy deploy files to remote server
/home/node/code/nextjs-template # zip -r .next.zip ./.next
/home/node/code/nextjs-template # zip -r public.zip ./public
λ scp .next.zip public.zip package.json package-lock.json .env.local user@winerva.com:/home/user/next-app
user@winerva.com's password:
.next.zip 100% 11MB 188.6KB/s
00:59
public.zip 100% 51MB 145.8KB/s
05:59
package.json 100% 1813 12.0KB/s
00:00
package-lock.json 100% 455KB 191.8KB/s
00:02
.env.local 100% 80 0.5KB/s
00:00
unzip deploy fiels to /var/www/next-app and use pm2 to run the Next.js App
//Unzip deploy files to /var/www/next-app folder
//change owner authorization
user@user-OptiPlex-9020M:~/next-app$ unzip .next.zip
user@user-OptiPlex-9020M:~/next-app$ unzip public.zip
user@user-OptiPlex-9020M:~/next-app$ npm install --omit-dev
user@user-OptiPlex-9020M:sudo mv ~/next-app/ /var/www/next-app
user@user-OptiPlex-9020M:sudo chown -R www-data:www-data /var/www/next-app
user@user-OptiPlex-9020M:sudo chmod -R 775 /var/www/next-app
//use pm2 to run
user@user-OptiPlex-9020M:cd /var/www/next-app
user@user-OptiPlex-9020M:pm2 start npm --name 'next-app' -- start
user@user-OptiPlex-9020M:pm2 save
user@user-OptiPlex-9020M:pm2 startup

Create a Nginx Configuration File And Restart Nginx to run
user@user-OptiPlex-9020M:~/next-app$ cat /etc/nginx/sites-available/next-app
server {
listen 80;
server_name www.winerva.com;
return 301 https://$host$request_uri;
}
server {
listen 443 ssl;
server_name www.winerva.com;
ssl_certificate /etc/letsencrypt/live/www.winerva.com/fullchain.pem;
ssl_certificate_key /etc/letsencrypt/live/www.winerva.com/privkey.pem;
add_header 'Access-Control-Allow-Origin' '*';
location / {
proxy_pass http://localhost:3000; # 轉發請求給 Next.js 應用
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection 'upgrade';
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header x-Forwarded-Proto $scheme;
}
# 設定日誌
error_log /var/log/nginx/next-app_error.log;
access_log /var/log/nginx/next-app_access.log;
}
user@user-OptiPlex-9020M: sudo ln -s /etc/nginx/sites-available/next-app /etc/nginx/sites-enabled/
user@user-OptiPlex-9020M:sudo systemctl restart nginx
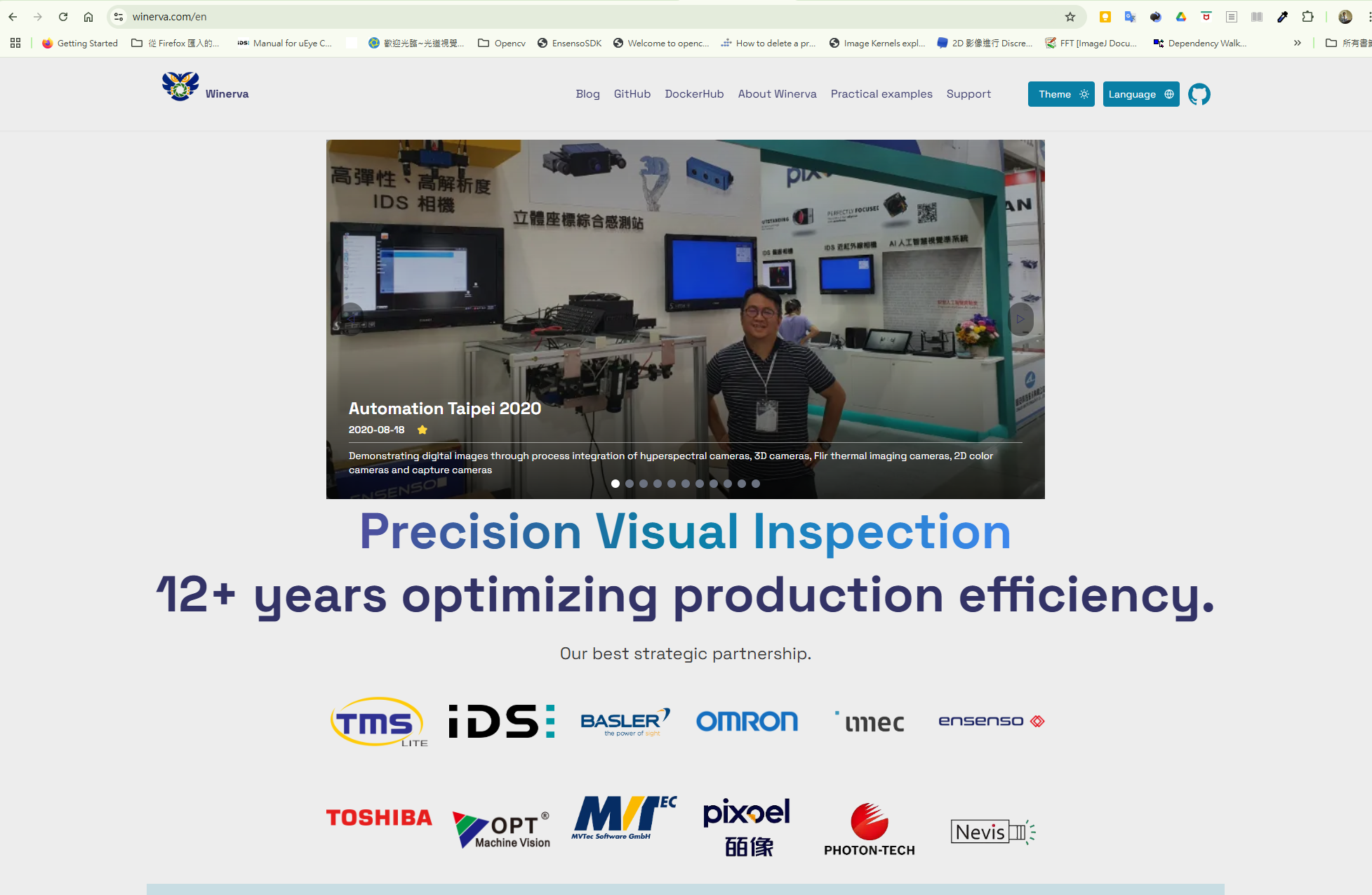