"testEnvironment": "node"
, which is appropriate for server-side tests"testEnvironment": "jsdom"
, which is appropriate for testing React components (which use the DOM) tests, This will allow Jest to simulate a browser environment to run your React component tests
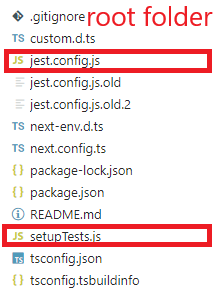
//setTest.js
import '@testing-library/jest-dom';
//jest.config.js
const nextJest = require("next/jest");
const createJestConfig = nextJest({});
const customJestConfig = {
moduleNameMapper: {
"^@/(.*)$": "<rootDir>/$1", // let recognize "@/..." path expression.
},
setupFilesAfterEnv: ['<rootDir>/setupTests.js'],
};
module.exports = createJestConfig(customJestConfig);
The following two Code snippets, one is seriver-side tests, and one is react componet tests.
dbConnect TestSuite is server side tests, use node for testEnvironment.
- afterEach: after each test function will do.
- afterAll: affter all test functions will do
- jest.clearAllMocks(): Clear all Jest mock function records.
- jest.restoreAllMocks(): Restore all mocks to restore the original function behavior
- jest.spyOn: Monitor whether a function is called (how many times, with what parameters)
- expect(spy).toHaveBeenCalled(): Check if the spy function is called
import dbConnect from "@/middleware/db-connect";
import mongoose from "mongoose";
import { MongoMemoryServer } from "mongodb-memory-server";
describe("dbConnect", () => {
let connection: any;
afterEach(async () => {
jest.clearAllMocks();
await connection.stop();
await mongoose.disconnect();
});
afterAll(async () => {
jest.restoreAllMocks();
})
test("calls MongoMemoryServer.create()", async () => {
const spy = jest.spyOn(MongoMemoryServer, "create");
connection = await dbConnect();
expect(spy).toHaveBeenCalled();
})
test("calls mongoose.disconnect()", async () => {
const spy = jest.spyOn(mongoose, "disconnect");
connection = await dbConnect();
expect(spy).toHaveBeenCalled();
})
test("calls mongoose.connect()", async () => {
const spy = jest.spyOn(mongoose, "connect");
connection = await dbConnect();
const MONGO_URI = connection.getUri();
expect(spy).toHaveBeenCalledWith(MONGO_URI, { dbName: "Weather" });
})
})
This React Unit Test file, it must be added the @jest-environment jsdom directive and import ‘@testing-library/jest-dom to tell jest use jsdom to run this test.
- render: use this function to render component
- screen: this object like browser concept.
- fireEvnet: you can use thie object to trigger event.
/**
* @jest-environment jsdom
*/
import { render, screen, fireEvent } from '@testing-library/react';
import '@testing-library/jest-dom';
import PageComponentWeather from '@/pages/components/weather';
describe("PageComponentWeather", () => {
test("renders correctly", () => {
render(<PageComponentWeather />);
//screen.debug()
expect(screen.getByText(/The weather is/i)).toBeInTheDocument();
expect(screen.getByText(/sunny/i)).toBeInTheDocument();
expect(screen.getByText(/and the counter shows/i)).toBeInTheDocument();
});
test("clicks the h1 element and updates the state", () => {
render(<PageComponentWeather />);
// 測試點擊 h1 元素
const h1Element = screen.getByText(/The weather is/i); // 你可以根據具體的文本修改
fireEvent.click(h1Element);
fireEvent.click(h1Element);
// 根據點擊後的效果進行驗證
expect(screen.getByText(/3/i)).toBeInTheDocument();
});
});
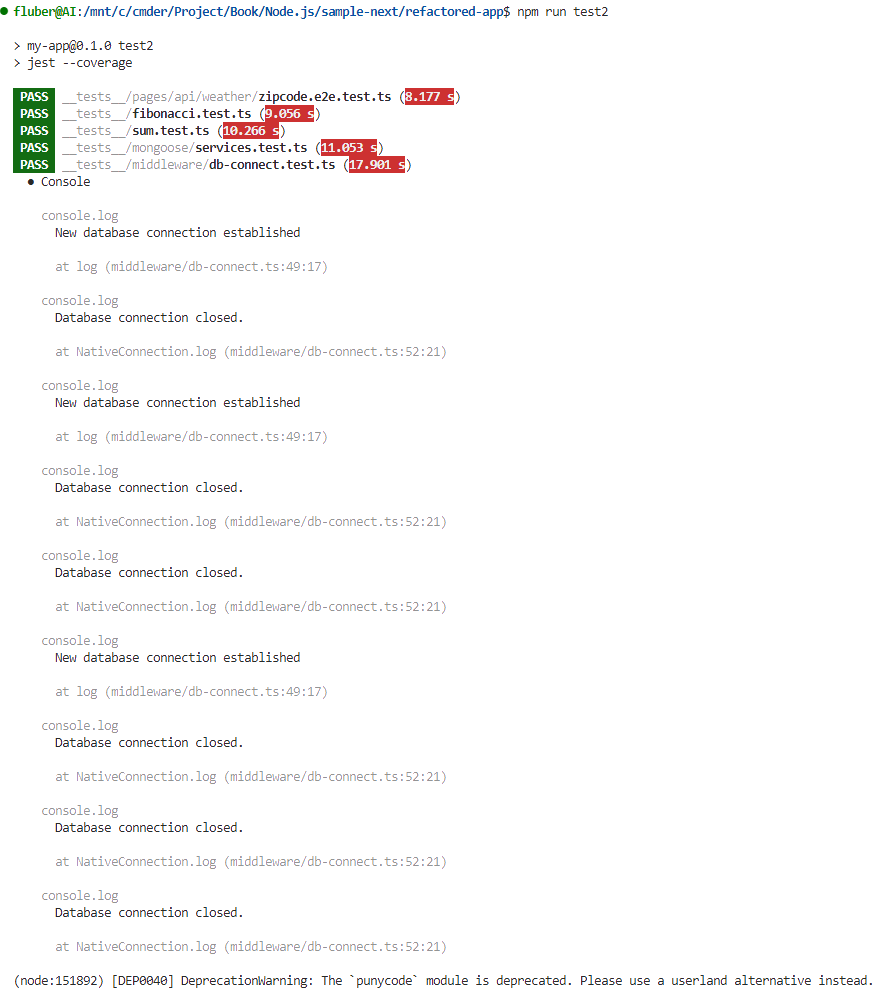
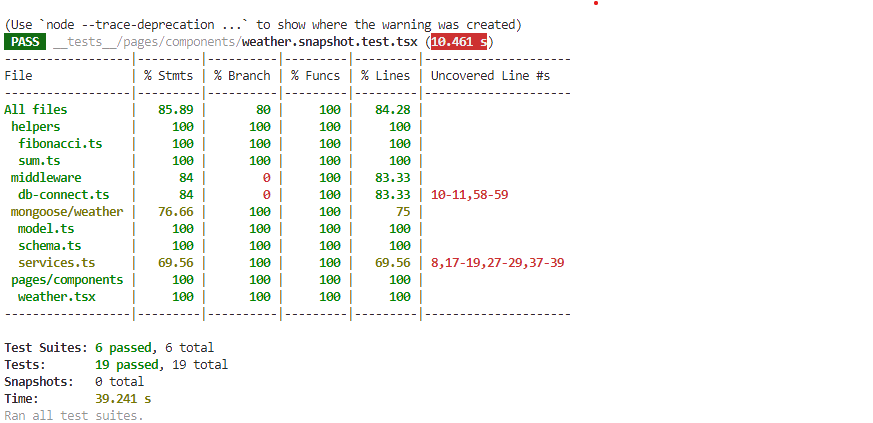