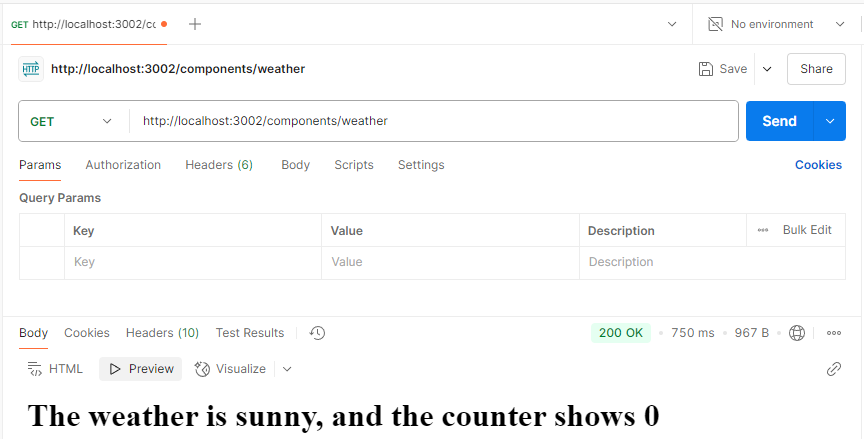
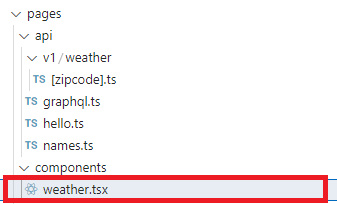
//weather.tsx
import type { NextPage } from "next";
import React, {useState, useEffect} from "react";
const PageComponentWeather: NextPage = () => {
const WeatherComponent = (props: WeatherProps) => {
const [count, setCount] = useState(0);
useEffect(() => {
setCount(1);
}, []);
return (
<h1 onClick={() => setCount(count + 1)}>
The weather is {props.weather},
and the counter shows {count}
</h1>
)
}
return (<WeatherComponent weather="sunny" />);
}
export default PageComponentWeather;
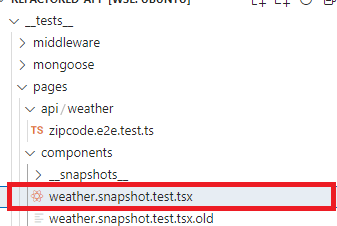
/**
* @jest-environment jsdom
*/
import { render, screen, fireEvent } from '@testing-library/react';
import '@testing-library/jest-dom';
import PageComponentWeather from '@/pages/components/weather';
describe("PageComponentWeather", () => {
test("renders correctly", () => {
render(<PageComponentWeather />);
expect(screen.getByText(/The weather is/i)).toBeInTheDocument();
expect(screen.getByText(/sunny/i)).toBeInTheDocument();
expect(screen.getByText(/and the counter shows/i)).toBeInTheDocument();
});
test("clicks the h1 element and updates the state", () => {
render(<PageComponentWeather />);
// 測試點擊 h1 元素
const h1Element = screen.getByText(/The weather is/i); // 你可以根據具體的文本修改
fireEvent.click(h1Element);
fireEvent.click(h1Element);
// 根據點擊後的效果進行驗證
expect(screen.getByText(/3/i)).toBeInTheDocument();
});
});
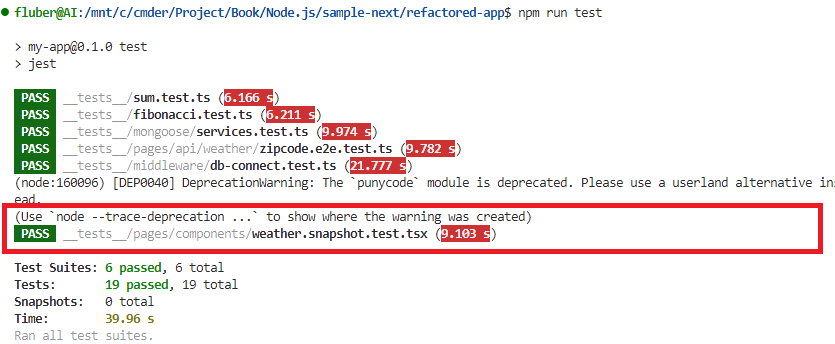