GraphQL has the built-in scale types Int, Float, String, Boolean, and ID. Exclamation marks (!) denote non-nullable fields, and lists within square brackets([ ]) indicate arrays.
//graphql schema
import gql from "graphql-tag";
//tagged template leteral
export const typeDefs = gql`
type LocationWeatherType {
zip: String!
weather: String!
tempC: String!
tempF: String!
friends: [String]!
}
input LocationWeatherInput {
zip: String!
weather: String
tempC: String
tempF: String
friends: [String]
}
type Query {
weather(zip: String): [LocationWeatherType]!
}
type Mutation {
weather(data: LocationWeatherInput): [LocationWeatherType]!
}
`;
//graphql resolvers
import { db } from "./data";
//use declare is to ensure that WeatherInterface is just a type definition and will not be translated into JavaScript code.
declare interface WeaterInterface {
zip: string;
weather: string;
tempC: string;
tempF: string;
friends: string[];
}
export const resolvers = {
Query: {
weather: async (_: any, param: WeaterInterface) => {
return [db.find((item) => item.zip === param.zip)]
}
},
Mutation: {
weather: async (_: any, param: {data: WeaterInterface}) => {
return [db.find((item) => item.zip == param.data.zip)];
}
}
}
- Create a graphql folder in the Next.js project root and put the schema.ts and resolvers.ts in the folder.
- Create a graphql.ts in the pages folder and implement the Graphql Server using ApolloServer.
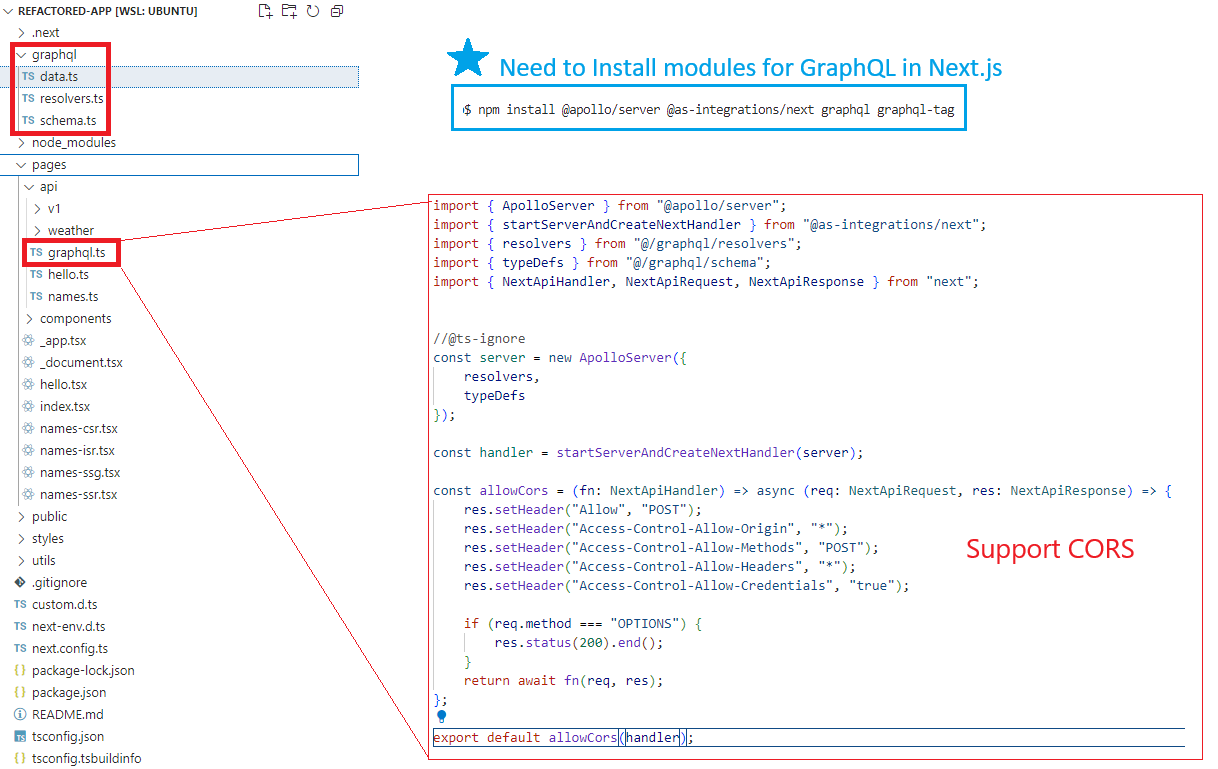
use browser http://localhost: 3000/api/graph to see the following screen. you can manipulation to query and mutation
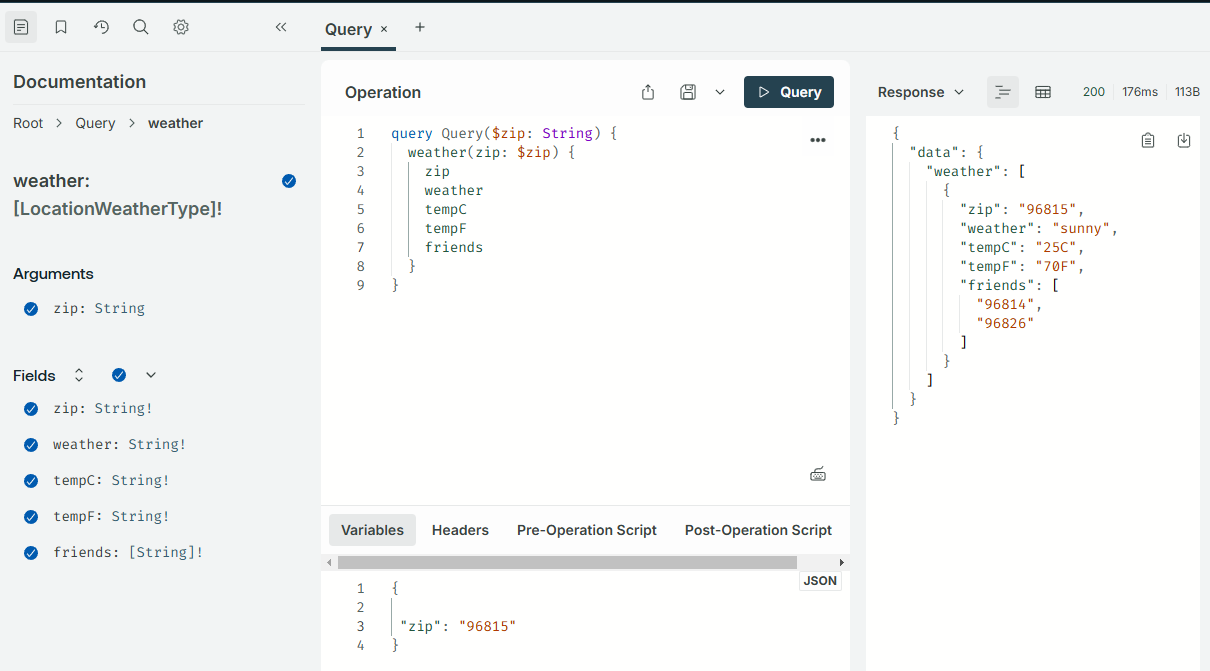
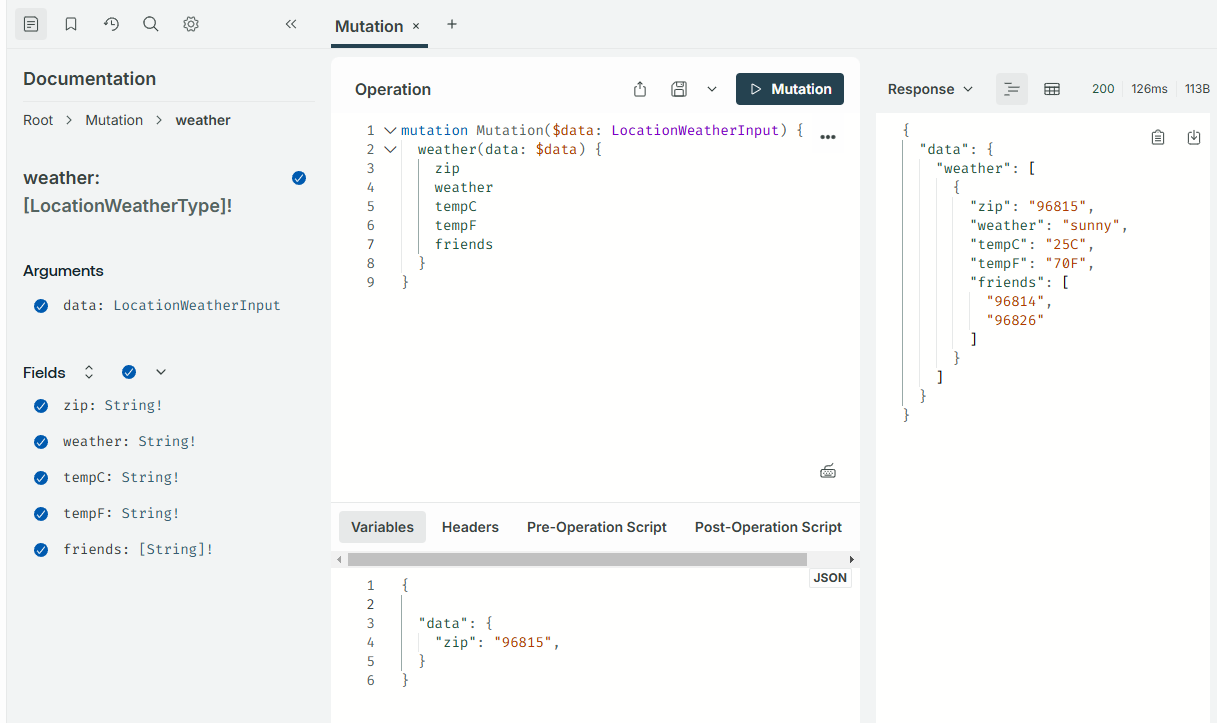