Node.js is a JavaScript runtime environment.
First, Install the Node.js in your computer system.
Windows:
- first go to the website, https://github.com/coreybutler/nvm-windows
- download and install nvm for windows
Linux:
- first go to the website, https://github.com/nvm-sh/nvm
- curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.40.1/install.sh | bash or wget -qO- https://raw.githubusercontent.com/nvm-sh/nvm/v0.40.1/install.sh | bash
- Running either of the above commands downloads a script and runs it. The script clones the nvm repository to
~/.nvm
, and attempts to add the source lines from the snippet below to the correct profile file (~/.bashrc
,~/.bash_profile
,~/.zshrc
, or~/.profile
). If you find the install script is updating the wrong profile file, set the$PROFILE
env var to the profile file’s path, and then rerun the installation script. export NVM_DIR="$([ -z "${XDG_CONFIG_HOME-}" ] && printf %s "${HOME}/.nvm" || printf %s "${XDG_CONFIG_HOME}/nvm")" [ -s "$NVM_DIR/nvm.sh" ] && . "$NVM_DIR/nvm.sh" # This loads nvm
use nvm to manage the different node version
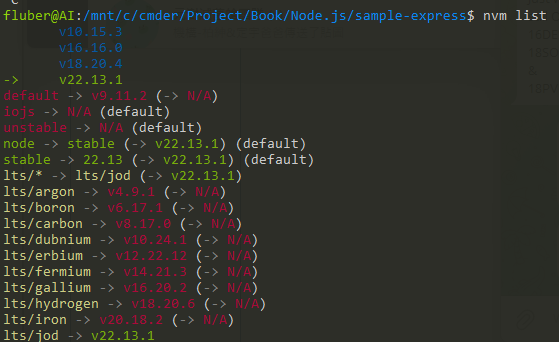
The default package manager for Node.js is npm.
NPM command
npm -v
npm init -y
npm install
npm uninstall
npm audit fix --force
npm fund
npm prune
npm update
npm init -y
will create a package.json and after install node.js module will generate a package-lock.json
package.json and package-lock.json will used by modules depandency manage.
package-lock.json tracking the exact version of every moduble and dependencies.
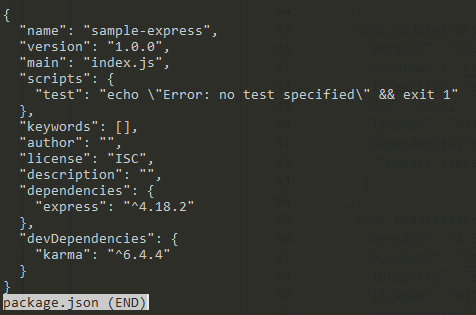
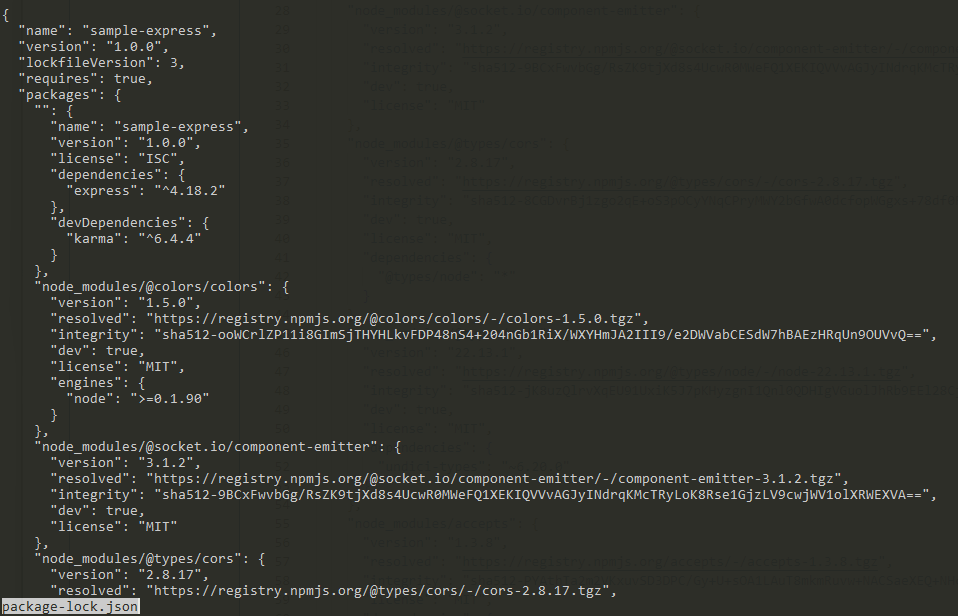
Create a Express.js application.
mkdir sample-express
cd sample-express
npm init -y
npm install express@4.18.2
vim index.js
------------------index.js-------------------
const express = require("express");
const server = express();
const port = 3000;
server.get("/", function(req, res) {
res.send("Hello World!");
});
server.listen(port, function() {
console.log("Listing on " + port);
});
--------------------------------------------
node index.js
Listing on 3000
NPX
when you installed Node.js, you also installed npx, which stands for node package execute.
npx jsonlint package.json // check valid json format.